前言
libuv总是报出一些让人难以理解的错误😂,作为一个C的项目,不具有Java、JavaScript、php那样的人气,很难百度到一些问题的答案,甚至google也不行。为了用好libuv,也为了学习吧。我开始看libuv的源码,不知道自己能走多远。。。
事件循环
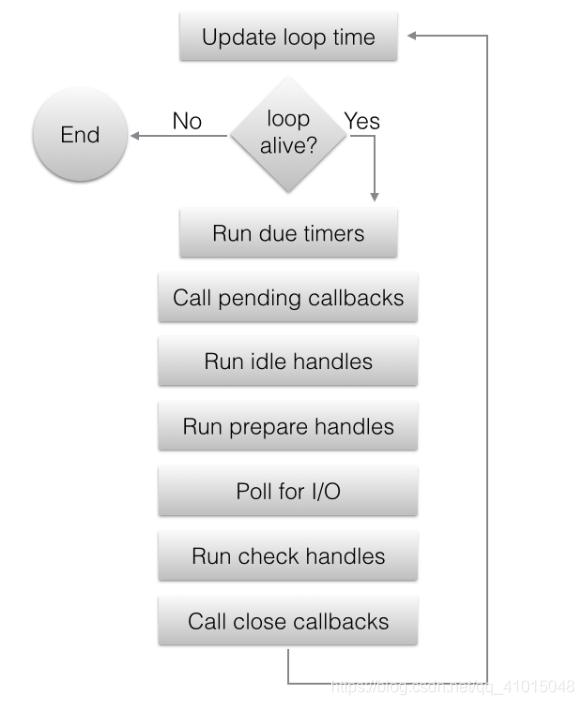
这是官方事件循环的示意图。链接->官方图片位置
1 | int uv_run(uv_loop_t* loop, uv_run_mode mode) { |
整个事件循环就是在主线程的uv_run()调用中执行的。我就跟着官方的介绍一步一步来看(官方介绍)。
第一步
The loop concept of ‘now’ is updated. The event loop caches the current time at the start of the event loop tick in order to reduce the number of time-related system calls.
第一步是更新时间。对应代码如下:
1 | uv__update_time(loop); |
总结来说就是调用这个函数,更新时间。uv__update_time实现我下一篇来介绍
第二步
If the loop is alive an iteration is started, otherwise the loop will exit immediately. So, when is a loop considered to be alive? If a loop has active and ref’d handles, active requests or closing handles it’s considered to be alive.
1 | r = uv__loop_alive(loop); |
用uv__loop_alive函数获取loop状态。
如果uv__loop_alive返回零或者loop->stop_flag == 1说明loop终止,直接跳过循环,到代码最下面(这里有一些性能的处理暂时不管 ),退出:
1 | /* The if statement lets gcc compile it to a conditional store. Avoids |
loop->stop_flag == 0的一个来源是调用了uv_stop,这个函数在手册中看见。它的源代码也很清晰。
1 | void uv_stop(uv_loop_t* loop) { |
如果loop状态OK,那么就进入循环中。
第三步
Due timers are run. All active timers scheduled for a time before the loop’s concept of now get their callbacks called.
对应代码这一部分:
1 | uv__run_timers(loop); |
将堆里面已经超时的拿出来运行。
第四步
Pending callbacks are called. All I/O callbacks are called right after polling for I/O, for the most part. There are cases, however, in which calling such a callback is deferred for the next loop iteration. If the previous iteration deferred any I/O callback it will be run at this point.
对应:
1 | ran_pending = uv__run_pending(loop); |
将loop->pending_queue中的任务拿出来运行。
第五、六、九步
5.Idle handle callbacks are called. Despite the unfortunate name, idle handles are run on every loop iteration, if they are active
6.Prepare handle callbacks are called. Prepare handles get their callbacks called right before the loop will block for I/O.
9.Check handle callbacks are called. Check handles get their callbacks called right after the loop has blocked for I/O. Check handles are essentially the counterpart of prepare handles.
1 | uv__run_idle(loop); |
这三部为什么要一起说呢?因为它们的实质是一样的。在每次循环固定的位置调用。
这三个函数定义在loop-watcher.c这个文件里面,它们是用宏定义定义的。只改了idle、prepare、check这三个名字的部分,其余部分函数都是一样的。
1 | /* Copyright Joyent, Inc. and other Node contributors. All rights reserved. |
第七步
Poll timeout is calculated. Before blocking for I/O the loop calculates for how long it should block. These are the rules when calculating the timeout:
If the loop was run with the UV_RUN_NOWAIT flag, the timeout is 0.
If the loop is going to be stopped (uv_stop() was called), the timeout is 0.
If there are no active handles or requests, the timeout is 0.
If there are any idle handles active, the timeout is 0.
If there are any handles pending to be closed, the timeout is 0.
If none of the above cases matches, the timeout of the closest timer is taken, or if there are no active timers, infinity.
1 | if ((mode == UV_RUN_ONCE && !ran_pending) || mode == UV_RUN_DEFAULT) |
这部分是取决于uv_run的模式的特殊处理,暂时不细看。
第八步
The loop blocks for I/O. At this point the loop will block for I/O for the duration calculated in the previous step. All I/O related handles that were monitoring a given file descriptor for a read or write operation get their callbacks called at this point.
1 | uv__io_poll(loop, timeout); |
这一部分对于不同操作系统有所不同,linux是poll,mac是kqueue。
第十步
Close callbacks are called. If a handle was closed by calling uv_close() it will get the close callback called.
1 | uv__run_closing_handles(loop); |
调用各类的close回调函数。
第十一、十二步
11.Special case in case the loop was run with UV_RUN_ONCE, as it implies forward progress. It’s possible that no I/O callbacks were fired after blocking for I/O, but some time has passed so there might be timers which are due, those timers get their callbacks called.
12.Iteration ends. If the loop was run with UV_RUN_NOWAIT or UV_RUN_ONCE modes the iteration ends and uv_run() will return. If the loop was run with UV_RUN_DEFAULT it will continue from the start if it’s still alive, otherwise it will also end.
对于uv_run不同模式的一点特殊处理。
1 | if (mode == UV_RUN_ONCE) { |
小结
宏观上梳理一下整个事件循环的过程。